Creating Reports With Data Filters Using Smart Report Engine
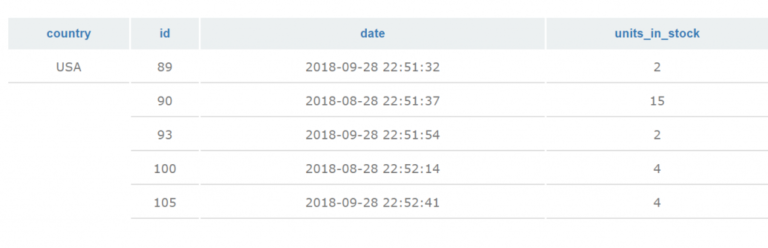
Before we begin, if you haven’t already done so, you might want to read the primary usage example. This post will help you understand how to start using Smart Report Engine.
In this tutorial, we will use Smart Report Engine to create a report based on a single table with data filters. Let’s assume we have an “items” table in a MYSQL DB. This table has valuable information about the available items in some sunglasses store, and we want to make a professional report based on that.
Report Options:
In this report we want to:
- Show only the following columns:
“id”,”product_name” , “country” , “units_in_stock”, “date_of_purchase” - Applying the following filters:
- Units in stock between 0 and 15
- Country is USA
- Date of purchase later than 2018-01-01
Please note that in a real-life scenario, the filtered value would be a variable defined in your project rather than a static value like “2018-01-01.”
We will start by showing the code in a native PHP project and then in a Laravel project.
1- Case of a native PHP Project
In a native PHP Project, the code will be something like the following:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | use SRE\Engine\CustomEngine; use SRE\Engine\ReportOptions; require_once "../sre_bootstrap.php" ; $report = new ReportOptions(SRE_PUBLIC_REPORT); $report ->select_tables( array ( "items" )) ->select_fields( array ( "id" , "country" , "date" , "units_in_stock" , "product_name" )) ->set_grouping( array ( "country" )) ->set_layout( "block" ) ->filter_like( "items" , "country" , "usa" ) ->filter_between( "items" , "units_in_stock" , 0, 15,SRE_NUMBER) $engine = new CustomEngine( $report ); $report_path = $engine ->create_report(); if ( $report_path ) { echo "Report created successfully! To visit your report please <a href='" . $report_path . "' /> click here </a> " ; } |
2- Case of Laravel Project
We will put the report generation logic in a service. It will be something like the following:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | namespace App\Services; use Sre\SmartReportingEngine\src\Engine\Constants; use Sre\SmartReportingEngine\src\Engine\CustomEngine; use Sre\SmartReportingEngine\src\Engine\ReportOptions; class ReportService { public function generatePublicReport() { $report = new ReportOptions(Constants::SRE_PUBLIC_REPORT); $report ->select_tables( array ( "items" )) ->select_fields( array ( "id" , "country" , "date" , "units_in_stock" , "product_name" )) ->set_grouping( array ( "country" )) ->set_layout( "block" ) ->filter_like( "items" , "country" , "usa" ) ->filter_between( "items" , "units_in_stock" , 0, 15,Constants::SRE_NUMBER) $engine = new CustomEngine( $report ); $report_path = $engine ->create_report(); return $report_path ; } } |
In the above code, we first set the needed options for this report using a “$report” object, which is an instance of the “ReportOptions” Class. Following are the report options defined in the example:
- Setting the Report as a “Public_Report”
A public report can be accessed freely without any integration with a login system. We pass the “SRE_PUBLIC_REPORT” flag to the constructor of the “ReportOptions” class to create a public report.
- Setting a specific MYSQL table as the data source of the report.
In the above example, we select a table with the name “items” as the data source of the report. To do this, we pass an array with only the “items” table to the “select_tables” method.
- Select some columns of the selected table to show in the report.
In the above example, we want to show only specific columns in the report. For this reason, we pass an array of the selected columns to the “select_fields” method.
- Applying data filters rules
In the above example, we apply two data filter rules; we do that by calling two filter methods, which are: “filter_like” to filter the “country” column, and “filter_between” to filter the “units_in_stock” column.
Please note that we can use the filter_between, filter_more, and filter_less methods with date and numeric data types.
- Grouping the report by a certain column
In the above example, we select a column with the name “country” for grouping the report. We do this by passing an array with only the “country” column to the “set_grouping” method.
Then after defining all the options for this report via the “ReportOptions” object, we will pass this object to the constructor of the “CustomEngine” class. By doing this, we create a new instance of the “CustomEngine” class; then, we used this new instance to call the “create_report” method to generate the report and return its URL.